DeepRL. todo: config the env and refactor
Showing
DeepRL/LICENSE
0 → 100644
DeepRL/assets/sprites/background-black.png
0 → 100644
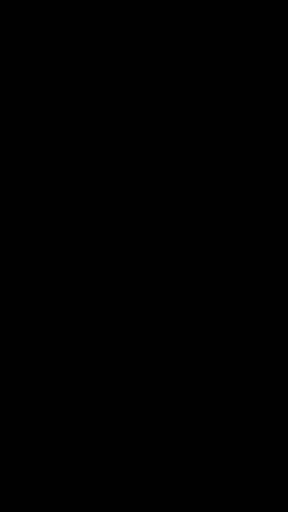
3.94 KB
DeepRL/assets/sprites/base.png
0 → 100644

664 Bytes
DeepRL/assets/sprites/pipe-green.png
0 → 100644

4.92 KB
DeepRL/assets/sprites/redbird-downflap.png
0 → 100644

2.88 KB
DeepRL/assets/sprites/redbird-midflap.png
0 → 100644

2.88 KB
DeepRL/assets/sprites/redbird-upflap.png
0 → 100644

2.88 KB
DeepRL/demo/flappybird.gif
0 → 100644
This diff is collapsed.
Click to expand it.
DeepRL/demo/flappybird.mp4
0 → 100644
File added
DeepRL/src/deep_q_network.py
0 → 100644
DeepRL/src/flappy_bird.py
0 → 100644
DeepRL/src/utils.py
0 → 100644
DeepRL/tensorboard/.gitkeep
0 → 100644
DeepRL/test.py
0 → 100644
DeepRL/train.py
0 → 100644
Please
register
or
sign in
to comment